[en] Don't show me the code
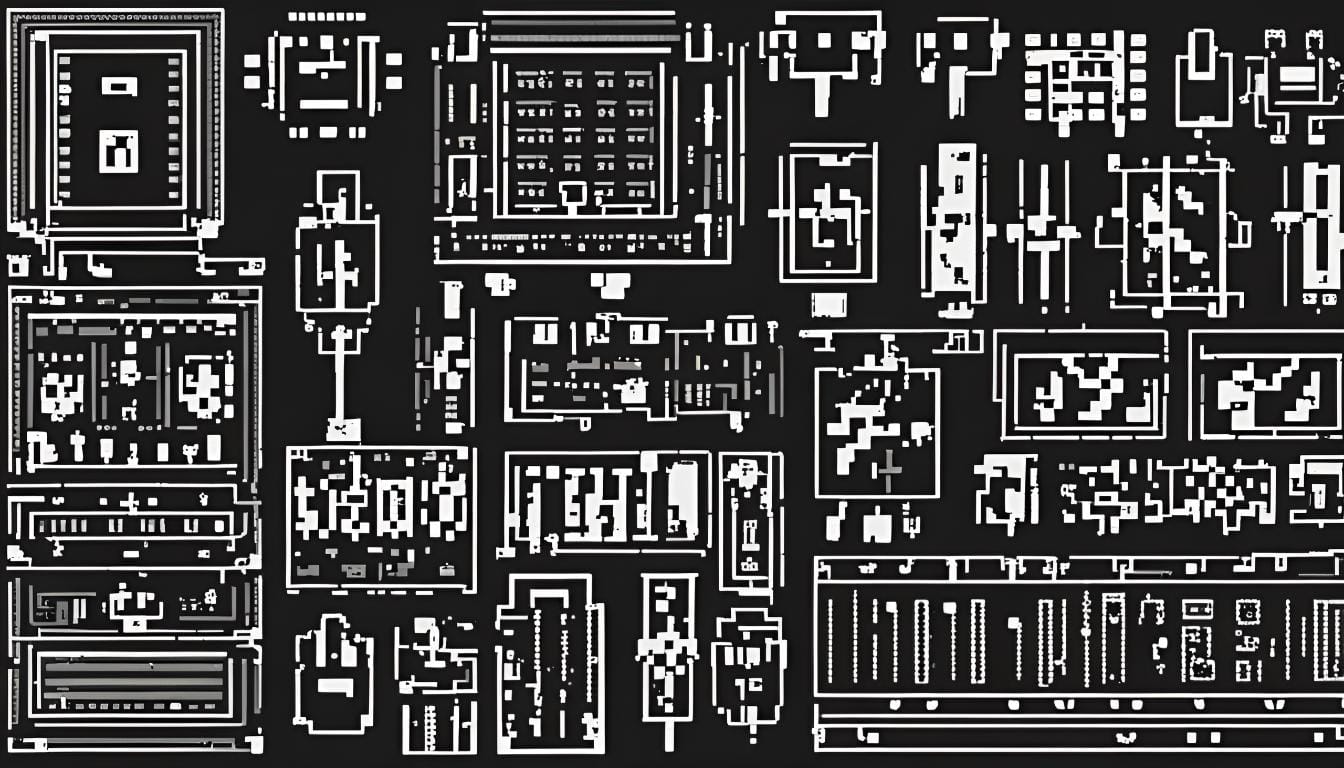
When we talk about blockchain security, the first thing that usually comes to mind is the smart contract code. And it makes sense: logical flaws, reentrancy, poor input validation, loose access control—any of these issues can turn a supposedly “bulletproof” contract into a minefield. It’s no surprise that, when analyzing the 100 biggest attacks, around 33% were caused by direct code vulnerabilities. This stat makes it crystal clear that audits, testing (including fuzzing, formal verification, static analysis, and manual review), adherence to established EIPs (like EIP-1967, EIP-2535), and a healthy dose of security paranoia are crucial to having a solid contract.
Another important data point: 27% of failures are linked to private key issues. It doesn’t matter if you use hot wallets (MetaMask, Phantom), cold wallets (Ledger, Trezor), or multisigs (Gnosis Safe): if key management is sloppy, an attacker doesn’t even need to crack your code. They just need to find (or leak) the keys. There was even a major protocol (I won’t name it here) that used a 3/11 threshold multisig, combining a weak security setup with careless key management. The result? Funds drained in no time. It’s pointless to have “bulletproof” code if your governance control is hanging out in the open.
Imagine a scenario: you have a governance smart contract with a well-implemented UUPS pattern (EIP-1967). You use OpenZeppelin’s AccessControl to keep permissions tidy, add a ReentrancyGuard to block malicious calls, and run a complete suite of tests with Foundry, including fuzz tests to spot weird behaviors. On paper, it’s all great.
// Simplified example. Do not use in production.
// SPDX-License-Identifier: unlicensed
pragma solidity ^0.8.19;
import {AccessControl} from "@openzeppelin/contracts/access/AccessControl.sol";
import {ReentrancyGuard} from "@openzeppelin/contracts/security/ReentrancyGuard.sol";
contract SecureGov is AccessControl, ReentrancyGuard {
bytes32 public constant UPGRADER_ROLE = keccak256("UPGRADER_ROLE");
address public implementation;
constructor(address admin) {
_setupRole(DEFAULT_ADMIN_ROLE, admin);
_setupRole(UPGRADER_ROLE, admin);
}
function upgradeContract(address newImplementation)
external
onlyRole(UPGRADER_ROLE)
nonReentrant
{
require(newImplementation != address(0), "Invalid address");
implementation = newImplementation;
}
}
But now consider the other side: who holds the admin or UPGRADER_ROLE? How are their corresponding keys protected? It’s all about layering security. Robust thresholds in multisigs (there’s no point using 3/11 just to “look good”), well-guarded keys—ideally in hardware wallets—and solid internal policies for key access and rotation are fundamental. Without this, your code might be impeccable, but the attacker slips right in through an open window and parties anyway.
And it doesn’t stop at key management. A holistic security approach also includes secure deployment practices, continuous integration and deployment (CI/CD) with verified pipelines, constant on-chain monitoring tools (logs, alerts for suspicious events), and staying tuned to the latest vulnerabilities reported by the community. Reading hack post-mortems, hanging out in security forums (like Ethereum Magicians), chatting in Discord with audit folks, or joining communities that analyze exploits (like ours) help keep your radar on high alert.
In short, approaching blockchain security goes beyond ensuring your smart contract is technically flawless. You have to consider the whole picture: from the development lifecycle, through deployment infrastructure, to key management, governance, and continuous operations. In the end, real security isn’t a fixed checklist—it’s a mindset. Understanding that if you only strengthen one part of the chain, you’re leaving a ready-made gap for someone to exploit is key. There’s no sugarcoating it: security is a multi-layered game—and you need to stay sharp on every single layer.